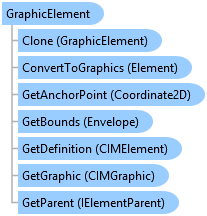
public class GraphicElement : Element, System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable<Element>
Public Class GraphicElement Inherits Element Implements System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable(Of Element)
Graphic elements are a type of Element and include inserted graphic points, lines, or area shapes. PictureElement and TextElement are also a type of graphic element.
The Graphic property returns a CIM representation of the graphic specific attributes for a GraphicElement. This may provide additional, finer grained properties exposed in the CIM that are not exposed in the managed API. SetGraphic applies the changes made to a modified CIMGraphic back to the GraphicElement.
The Clone method allows you to duplicate existing graphic elements on your page layout. This can be useful where you may have a variable number of pictures, for example, on each page in the map series. Rather than authoring a layout with all possibilities, a single picture element can be cloned and updated the appropriate number of times to reference a different set of pictures on disk for each page in the series.
//This example references a graphic element on a layout and sets its Transparency property (which is not available in the managed API) //by accessing the element's CIMGraphic. //Added references using ArcGIS.Core.CIM; //CIM using ArcGIS.Desktop.Core; //Project using ArcGIS.Desktop.Layouts; //Layout class using ArcGIS.Desktop.Framework.Threading.Tasks; //QueuedTask public class GraphicElementExample1 { public static Task<bool> UpdateElementTransparencyAsync(string LayoutName, string ElementName, int TransValue) { //Reference a layoutitem in a project by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals(LayoutName)); if (layoutItem == null) return Task.FromResult(false); return QueuedTask.Run<bool>(() => { //Reference and load the layout associated with the layout item Layout lyt = layoutItem.GetLayout(); //Reference a element by name GraphicElement graElm = lyt.FindElement(ElementName) as GraphicElement; if (graElm == null) return false; //Modify the Transparency property that exists only in the CIMGraphic class. CIMGraphic CIMGra = graElm.Graphic as CIMGraphic; CIMGra.Transparency = TransValue; //e.g., TransValue = 50 graElm.SetGraphic(CIMGra); return true; }); } }
//This example finds a layout element by name and clones it a specified number of times and applies an accumlative offset for each //cloned element. //Added references using ArcGIS.Core.CIM; //CIM using ArcGIS.Desktop.Core; //Project using ArcGIS.Desktop.Layouts; //Layout class using ArcGIS.Desktop.Framework.Threading.Tasks; //QueuedTask public class GraphicElementExample2 { public static Task<bool> CloneElementAsync(string LayoutName, string ElementName, double offset, int numCopies) { //Reference a layoutitem in a project by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals(LayoutName)); if (layoutItem == null) return Task.FromResult(false); return QueuedTask.Run<bool>(() => { //Reference and load the layout associated with the layout item Layout lyt = layoutItem.GetLayout(); //Reference a element by name GraphicElement graElm = lyt.FindElement(ElementName) as GraphicElement; if (graElm == null) return false; //Loop through the number of copies, clone, and set the offsets for each new element double orig_offset = offset; while (numCopies != 0) { GraphicElement cloneElm = graElm.Clone("Clone"); cloneElm.SetX(cloneElm.GetX() + offset); cloneElm.SetY(cloneElm.GetY() - offset); offset = offset + orig_offset; numCopies = numCopies - 1; } return true; }); } }
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Layouts.Element
ArcGIS.Desktop.Layouts.GraphicElement
ArcGIS.Desktop.Layouts.PictureElement
ArcGIS.Desktop.Layouts.TextElement
Target Platforms: Windows 10, Windows 8.1, Windows 7