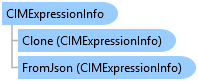
public class CIMExpressionInfo : CIMObject, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMExpressionInfo Inherits CIMObject Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
//Consult https://github.com/Esri/arcade-expressions/ and //https://developers.arcgis.com/arcade/ for more examples //and arcade reference QueuedTask.Run(() => { //construct an expression var query = @"Count($layer)";//count of features in "layer" //construct a CIMExpressionInfo var arcade_expr = new CIMExpressionInfo() { Expression = query.ToString(), //Return type can be string, numeric, or default //When set to default, addin is responsible for determining //the return type ReturnType = ExpressionReturnType.Default }; //Construct an evaluator //select the relevant profile - it must support Pro and it must //contain any profile variables you are using in your expression. //Consult: https://developers.arcgis.com/arcade/profiles/ using (var arcade = ArcadeScriptEngine.Instance.CreateEvaluator( arcade_expr, ArcadeProfile.Popups)) { //Provision values for any profile variables referenced... //in our case '$layer' var variables = new List<KeyValuePair<string, object>>() { new KeyValuePair<string, object>("$layer", featLayer) }; //evaluate the expression try { var result = arcade.Evaluate(variables).GetResult(); System.Diagnostics.Debug.WriteLine($"Result: {result.ToString()}"); } //handle any exceptions catch (InvalidProfileVariableException ipe) { //something wrong with the profile variable specified //TODO... } catch (EvaluationException ee) { //something wrong with the query evaluation //TODO... } } });
//Consult https://github.com/Esri/arcade-expressions/ and //https://developers.arcgis.com/arcade/ for more examples //and arcade reference QueuedTask.Run(() => { //construct an expression var query = @"$feature.AreaInAcres * 43560.0";//convert acres to ft 2 //construct a CIMExpressionInfo var arcade_expr = new CIMExpressionInfo() { Expression = query.ToString(), //Return type can be string, numeric, or default //When set to default, addin is responsible for determining //the return type ReturnType = ExpressionReturnType.Default }; //Construct an evaluator //select the relevant profile - it must support Pro and it must //contain any profile variables you are using in your expression. //Consult: https://developers.arcgis.com/arcade/profiles/ using (var arcade = ArcadeScriptEngine.Instance.CreateEvaluator( arcade_expr, ArcadeProfile.Popups)) { //we are evaluating the expression against all features using (var rc = featLayer.Search()) { while (rc.MoveNext()) { //Provision values for any profile variables referenced... //in our case '$feature' var variables = new List<KeyValuePair<string, object>>() { new KeyValuePair<string, object>("$feature", rc.Current) }; //evaluate the expression (per feature in this case) try { var result = arcade.Evaluate(variables).GetResult(); var val = ((double)result).ToString("0.0#"); System.Diagnostics.Debug.WriteLine( $"{rc.Current.GetObjectID()} area: {val} ft2"); } //handle any exceptions catch (InvalidProfileVariableException ipe) { //something wrong with the profile variable specified //TODO... } catch (EvaluationException ee) { //something wrong with the query evaluation //TODO... } } } } });
var lyr = MapView.Active.Map.GetLayersAsFlattenedList().OfType<FeatureLayer>().FirstOrDefault(f => f.ShapeType == esriGeometryType.esriGeometryPolygon); if (lyr == null) return; QueuedTask.Run(() => { // GetRenderer from Layer (assumes it is a unique value renderer) var uvRenderer = lyr.GetRenderer() as CIMUniqueValueRenderer; if (uvRenderer == null) return; //layer has STATE_NAME field //community sample Data\Admin\AdminSample.aprx string expression = "if ($view.scale > 21000000) { return $feature.STATE_NAME } else { return 'All' }"; CIMExpressionInfo updatedExpressionInfo = new CIMExpressionInfo { Expression = expression, Title = "Custom" // can be any string used for UI purpose. }; //set the renderer's expression uvRenderer.ValueExpressionInfo = updatedExpressionInfo; //SetRenderer on Layer lyr.SetRenderer(uvRenderer); });
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMExpressionInfo
Target Platforms: Windows 11, Windows 10