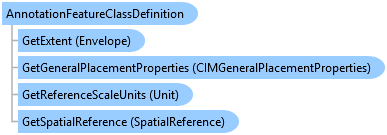
public sealed class AnnotationFeatureClassDefinition : ArcGIS.Core.Data.FeatureClassDefinition, System.IDisposable
Public NotInheritable Class AnnotationFeatureClassDefinition Inherits ArcGIS.Core.Data.FeatureClassDefinition Implements System.IDisposable
// get an anno layer AnnotationLayer annoLayer = MapView.Active.Map.GetLayersAsFlattenedList().OfType<AnnotationLayer>().FirstOrDefault(); if (annoLayer == null) return; QueuedTask.Run(() => { Inspector insp = null; // get the anno feature class var fc = annoLayer.GetFeatureClass() as ArcGIS.Core.Data.Mapping.AnnotationFeatureClass; // get the featureclass CIM definition which contains the labels, symbols var cimDefinition = fc.GetDefinition() as ArcGIS.Core.Data.Mapping.AnnotationFeatureClassDefinition; var labels = cimDefinition.GetLabelClassCollection(); var symbols = cimDefinition.GetSymbolCollection(); // make sure there are labels, symbols if ((labels.Count == 0) || (symbols.Count == 0)) return; // find the label class required // typically you would use a subtype name or some other characteristic // in this case lets just use the first one var label = labels[0]; // each label has a textSymbol // the symbolName *should* be the symbolID to be used var symbolName = label.TextSymbol.SymbolName; int symbolID = -1; if (!int.TryParse(symbolName, out symbolID)) { // int.TryParse fails - attempt to find the symbolName in the symbol collection foreach (var symbol in symbols) { if (symbol.Name == symbolName) { symbolID = symbol.ID; break; } } } // no symbol? if (symbolID == -1) return; // load the schema insp = new Inspector(); insp.LoadSchema(annoLayer); // ok to assign these fields using the inspector[fieldName] methodology // these fields are guaranteed to exist in the annotation schema insp["AnnotationClassID"] = label.ID; insp["SymbolID"] = symbolID; // set up some additional annotation properties AnnotationProperties annoProperties = insp.GetAnnotationProperties(); annoProperties.FontSize = 36; annoProperties.TextString = "My Annotation feature"; annoProperties.VerticalAlignment = VerticalAlignment.Top; annoProperties.HorizontalAlignment = HorizontalAlignment.Justify; insp.SetAnnotationProperties(annoProperties); var tags = new[] { "Annotation", "tag1", "tag2" }; // use daml-id rather than guid string defaultTool = "esri_editing_SketchStraightAnnoTool"; // tool filter is the tools to filter OUT var toolFilter = new[] { "esri_editing_SketchCurvedAnnoTool" }; // create a new template var newTemplate = annoLayer.CreateTemplate("new anno template", "description", insp, defaultTool, tags, toolFilter); });
public async Task CreatingAnAnnotationFeature(Geodatabase geodatabase) { await ArcGIS.Desktop.Framework.Threading.Tasks.QueuedTask.Run(() => { using (AnnotationFeatureClass annotationFeatureClass = geodatabase.OpenDataset<AnnotationFeatureClass>("Annotation // feature // class // name")) using (AnnotationFeatureClassDefinition annotationFeatureClassDefinition = annotationFeatureClass.GetDefinition()) using (RowBuffer rowBuffer = annotationFeatureClass.CreateRowBuffer()) using (AnnotationFeature annotationFeature = annotationFeatureClass.CreateRow(rowBuffer)) { annotationFeature.SetAnnotationClassID(0); annotationFeature.SetStatus(AnnotationStatus.Placed); // Get the annotation labels from the label collection IReadOnlyList<CIMLabelClass> labelClasses = annotationFeatureClassDefinition.GetLabelClassCollection(); // Setup the symbol reference with the symbol id and the text symbol CIMSymbolReference cimSymbolReference = new CIMSymbolReference(); cimSymbolReference.Symbol = labelClasses[0].TextSymbol.Symbol; cimSymbolReference.SymbolName = labelClasses[0].TextSymbol.SymbolName; // Setup the text graphic CIMTextGraphic cimTextGraphic = new CIMTextGraphic(); cimTextGraphic.Text = "Charlotte, North Carolina"; cimTextGraphic.Shape = new MapPointBuilderEx(new Coordinate2D(-80.843, 35.234), SpatialReferences.WGS84).ToGeometry(); cimTextGraphic.Symbol = cimSymbolReference; // Set the symbol reference on the graphic and store annotationFeature.SetGraphic(cimTextGraphic); annotationFeature.Store(); } }); }
System.Object
ArcGIS.Core.CoreObjectsBase
ArcGIS.Core.Data.Definition
ArcGIS.Core.Data.TableDefinition
ArcGIS.Core.Data.FeatureClassDefinition
ArcGIS.Core.Data.Mapping.AnnotationFeatureClassDefinition
Target Platforms: Windows 11, Windows 10