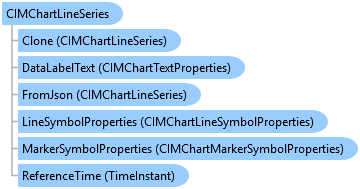
public class CIMChartLineSeries : CIMChartSeries, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMChartLineSeries Inherits CIMChartSeries Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
// For more information on the chart CIM specification: // https://github.com/Esri/cim-spec/blob/main/docs/v3/CIMCharts.md // Define fields names used in chart parameters. const string dateField = "last_review"; const string numericField = "price"; var lyrsLine = MapView.Active.Map.GetLayersAsFlattenedList().OfType<FeatureLayer>(); var lyrLine = lyrsLine.First(); var lyrDefLine = lyrLine.GetDefinition(); // Define line chart CIM properties var lineChart = new CIMChart { Name = "lineChart", GeneralProperties = new CIMChartGeneralProperties { Title = $"Line chart for {dateField} summarized by {numericField}", UseAutomaticTitle = false }, Series = new CIMChartSeries[] { new CIMChartLineSeries { UniqueName = "lineChartSeries", Name = $"Sum({numericField})", // Specify date field and numeric field Fields = new string[] { dateField, numericField }, // Specify aggregation type FieldAggregation = new string[] { string.Empty, "SUM" }, // Specify custom time bin of 6 months TimeAggregationType = ChartTimeAggregationType.EqualIntervalsFromStartTime, TimeIntervalSize = 6.0, TimeIntervalUnits = esriTimeUnits.esriTimeUnitsMonths, // NOTE: When setting custom time binning, be sure to set CalculateAutomaticTimeInterval = false CalculateAutomaticTimeInterval = false, // Define custom line color ColorType = ChartColorType.CustomColor, LineSymbolProperties = new CIMChartLineSymbolProperties { Style = ChartLineDashStyle.DashDot, Color = new CIMRGBColor { R = 0, G = 150, B = 20 }, }, MarkerSymbolProperties = new CIMChartMarkerSymbolProperties { Color = new CIMRGBColor { R = 0, G = 150, B = 20 } } }, } }; // Add new chart to layer's existing list of charts (if any exist) var newChartsLine = new CIMChart[] { lineChart }; var allChartsLine = (lyrDefLine == null) ? newChartsLine : lyrDefLine.Charts.Concat(newChartsLine); // Add CIM chart to layer defintion lyrDefLine.Charts = allChartsLine.ToArray(); lyrLine.SetDefinition(lyrDefLine);
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMChartSeries
ArcGIS.Core.CIM.CIMChartLineSeries
Target Platforms: Windows 11, Windows 10