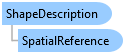
public sealed class ShapeDescription : Description
Public NotInheritable Class ShapeDescription Inherits Description
FieldDescription bigIntegerFieldDescription = new FieldDescription("BigInteger_64", FieldType.BigInteger);
// 64-bit FieldDescription oidFieldDescription_64 = new FieldDescription("ObjectID_64", FieldType.OID) { Length = 8 }; // 32-bit FieldDescription oidFieldDescription_32 = new FieldDescription("ObjectID_32", FieldType.OID) { Length = 4 };
// Earthquake occurrences date and time // 9/28/2014 (DateOnly) FieldDescription earthquakeDateOnlyFieldDescription = new FieldDescription("Earthquake_DateOnly", FieldType.DateOnly); // 1:16:42 AM (TimeOnly) FieldDescription earthquakeTimeOnlyFieldDescription = new FieldDescription("Earthquake_TimeOnly", FieldType.TimeOnly); // 9/28/2014 1:16:42.000 AM -09:00 (Timestamp with Offset) FieldDescription earthquakeTimestampOffsetFieldDescription = new FieldDescription("Earthquake_TimestampOffset_Local", FieldType.TimestampOffset); // 9/28/2014 1:16:42 AM (DateTime) FieldDescription earthquakeDateFieldDescription = new FieldDescription("Earthquake_Date", FieldType.Date);
public void CreateFeatureDatasetWithFeatureClassSnippet(Geodatabase geodatabase) { // Creating a FeatureDataset named as 'Parcel_Information' and a FeatureClass with name 'Parcels' in one operation string featureDatasetName = "Parcel_Information"; string featureClassName = "Parcels"; SchemaBuilder schemaBuilder = new SchemaBuilder(geodatabase); // Create a FeatureDataset token FeatureDatasetDescription featureDatasetDescription = new FeatureDatasetDescription(featureDatasetName, SpatialReferences.WGS84); FeatureDatasetToken featureDatasetToken = schemaBuilder.Create(featureDatasetDescription); // Create a FeatureClass description FeatureClassDescription featureClassDescription = new FeatureClassDescription(featureClassName, new List<FieldDescription>() { new FieldDescription("Id", FieldType.Integer), new FieldDescription("Address", FieldType.String) }, new ShapeDescription(GeometryType.Point, SpatialReferences.WGS84)); // Create a FeatureClass inside a FeatureDataset FeatureClassToken featureClassToken = schemaBuilder.Create(new FeatureDatasetDescription(featureDatasetToken), featureClassDescription); // Build status bool buildStatus = schemaBuilder.Build(); // Build errors if (!buildStatus) { IReadOnlyList<string> errors = schemaBuilder.ErrorMessages; } }
public void CreateFeatureClassInsideFeatureDatasetSnippet(Geodatabase geodatabase) { // Creating a FeatureClass named as 'Tax_Jurisdiction' in existing FeatureDataset with name 'Parcels_Information' string featureDatasetName = "Parcels_Information"; string featureClassName = "Tax_Jurisdiction"; // Create a FeatureClass description FeatureClassDescription featureClassDescription = new FeatureClassDescription(featureClassName, new List<FieldDescription>() { new FieldDescription("Tax_Id", FieldType.Integer), new FieldDescription("Address", FieldType.String) }, new ShapeDescription(GeometryType.Point, SpatialReferences.WGS84)); FeatureDatasetDefinition featureDatasetDefinition = geodatabase.GetDefinition<FeatureDatasetDefinition>(featureDatasetName); SchemaBuilder schemaBuilder = new SchemaBuilder(geodatabase); // Create a FeatureClass inside a FeatureDataset using a FeatureDatasetDefinition schemaBuilder.Create(new FeatureDatasetDescription(featureDatasetDefinition), featureClassDescription); // Build status bool buildStatus = schemaBuilder.Build(); // Build errors if (!buildStatus) { IReadOnlyList<string> errors = schemaBuilder.ErrorMessages; } }
public void CreateStandAloneAnnotationFeatureClass(Geodatabase geodatabase, SpatialReference spatialReference) { // Creating a Cities annotation feature class // with following user defined fields // Name // GlobalID // Annotation feature class name string annotationFeatureClassName = "CitiesAnnotation"; // Create user defined attribute fields for annotation feature class FieldDescription globalIDFieldDescription = FieldDescription.CreateGlobalIDField(); FieldDescription nameFieldDescription = FieldDescription.CreateStringField("Name", 255); // Create a list of all field descriptions List<FieldDescription> fieldDescriptions = new List<FieldDescription> { globalIDFieldDescription, nameFieldDescription }; // Create a ShapeDescription object ShapeDescription shapeDescription = new ShapeDescription(GeometryType.Polygon, spatialReference); // Create general placement properties for Maplex engine CIMMaplexGeneralPlacementProperties generalPlacementProperties = new CIMMaplexGeneralPlacementProperties { AllowBorderOverlap = true, PlacementQuality = MaplexQualityType.High, DrawUnplacedLabels = true, InvertedLabelTolerance = 1.0, RotateLabelWithDisplay = true, UnplacedLabelColor = new CIMRGBColor { R = 0, G = 255, B = 0, Alpha = 0.5f // Green } }; // Create general placement properties for Standard engine //CIMStandardGeneralPlacementProperties generalPlacementProperties = // new CIMStandardGeneralPlacementProperties // { // DrawUnplacedLabels = true, // InvertedLabelTolerance = 3.0, // RotateLabelWithDisplay = true, // UnplacedLabelColor = new CIMRGBColor // { // R = 255, G = 0, B = 0, Alpha = 0.5f // Red // } // }; // Create annotation label classes // Green label CIMLabelClass greenLabelClass = new CIMLabelClass { Name = "Green", ExpressionTitle = "Expression-Green", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 1, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Tahoma", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 255, 0) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Green" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Blue label CIMLabelClass blueLabelClass = new CIMLabelClass { Name = "Blue", ExpressionTitle = "Expression-Blue", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 2, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Consolas", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 0, 255) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Blue" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Create a list of labels List<CIMLabelClass> labelClasses = new List<CIMLabelClass> { greenLabelClass, blueLabelClass }; // Create an annotation feature class description object to describe the feature class to create AnnotationFeatureClassDescription annotationFeatureClassDescription = new AnnotationFeatureClassDescription(annotationFeatureClassName, fieldDescriptions, shapeDescription, generalPlacementProperties, labelClasses) { IsAutoCreate = true, IsSymbolIDRequired = false, IsUpdatedOnShapeChange = true }; // Create a SchemaBuilder object SchemaBuilder schemaBuilder = new SchemaBuilder(geodatabase); // Add the creation of the Cities annotation feature class to the list of DDL tasks schemaBuilder.Create(annotationFeatureClassDescription); // Execute the DDL bool success = schemaBuilder.Build(); // Inspect error messages if (!success) { IReadOnlyList<string> errorMessages = schemaBuilder.ErrorMessages; //etc. } }
public void CreateFeatureLinkedAnnotationFeatureClass(Geodatabase geodatabase, SpatialReference spatialReference) { // Creating a feature-linked annotation feature class between water pipe and valve in water distribution network // with following user defined fields // PipeName // GlobalID // Annotation feature class name string annotationFeatureClassName = "WaterPipeAnnotation"; // Create user defined attribute fields for annotation feature class FieldDescription pipeGlobalID = FieldDescription.CreateGlobalIDField(); FieldDescription nameFieldDescription = FieldDescription.CreateStringField("Name", 255); // Create a list of all field descriptions List<FieldDescription> fieldDescriptions = new List<FieldDescription> { pipeGlobalID, nameFieldDescription }; // Create a ShapeDescription object ShapeDescription shapeDescription = new ShapeDescription(GeometryType.Polygon, spatialReference); // Create general placement properties for Maplex engine CIMMaplexGeneralPlacementProperties generalPlacementProperties = new CIMMaplexGeneralPlacementProperties { AllowBorderOverlap = true, PlacementQuality = MaplexQualityType.High, DrawUnplacedLabels = true, InvertedLabelTolerance = 1.0, RotateLabelWithDisplay = true, UnplacedLabelColor = new CIMRGBColor { R = 255, G = 0, B = 0, Alpha = 0.5f } }; // Create annotation label classes // Green label CIMLabelClass greenLabelClass = new CIMLabelClass { Name = "Green", ExpressionTitle = "Expression-Green", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 1, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Tahoma", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 255, 0) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Green" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Blue label CIMLabelClass blueLabelClass = new CIMLabelClass { Name = "Blue", ExpressionTitle = "Expression-Blue", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 2, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Consolas", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 0, 255) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Blue" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Create a list of labels List<CIMLabelClass> labelClasses = new List<CIMLabelClass> { greenLabelClass, blueLabelClass }; // Create linked feature description // Linked feature class name string linkedFeatureClassName = "WaterPipe"; // Create fields for water pipe FieldDescription waterPipeGlobalID = FieldDescription.CreateGlobalIDField(); FieldDescription pipeName = FieldDescription.CreateStringField("PipeName", 255); // Create a list of water pipe field descriptions List<FieldDescription> pipeFieldDescriptions = new List<FieldDescription> { waterPipeGlobalID, pipeName }; // Create a linked feature class description FeatureClassDescription linkedFeatureClassDescription = new FeatureClassDescription(linkedFeatureClassName, pipeFieldDescriptions, new ShapeDescription(GeometryType.Polyline, spatialReference)); // Create a SchemaBuilder object SchemaBuilder schemaBuilder = new SchemaBuilder(geodatabase); // Add the creation of the linked feature class to the list of DDL tasks FeatureClassToken linkedFeatureClassToken = schemaBuilder.Create(linkedFeatureClassDescription); // Create an annotation feature class description object to describe the feature class to create AnnotationFeatureClassDescription annotationFeatureClassDescription = new AnnotationFeatureClassDescription(annotationFeatureClassName, fieldDescriptions, shapeDescription, generalPlacementProperties, labelClasses, new FeatureClassDescription(linkedFeatureClassToken)) { IsAutoCreate = true, IsSymbolIDRequired = false, IsUpdatedOnShapeChange = true }; // Add the creation of the annotation feature class to the list of DDL tasks schemaBuilder.Create(annotationFeatureClassDescription); // Execute the DDL bool success = schemaBuilder.Build(); // Inspect error messages if (!success) { IReadOnlyList<string> errorMessages = schemaBuilder.ErrorMessages; //etc. } }
System.Object
ArcGIS.Core.Data.DDL.Description
ArcGIS.Core.Data.DDL.ShapeDescription
Target Platforms: Windows 11, Windows 10