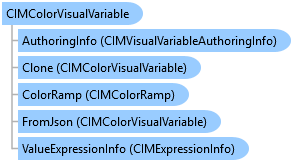
public class CIMColorVisualVariable : CIMVisualVariable, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMColorVisualVariable Inherits CIMVisualVariable Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
//Consult https://github.com/Esri/arcade-expressions/ and //https://developers.arcgis.com/arcade/ for more examples //and arcade reference var mv = MapView.Active; var map = mv.Map; QueuedTask.Run(() => { //Assume we a layer - Oregon County (poly) that is using Visual Variable //expressions that we want to evaluate interactively... var def = oregon_cnts.GetDefinition() as CIMFeatureLayer; //Most all feature renderers have a VisualVariable collection var renderer = def.Renderer as CIMUniqueValueRenderer; var vis_variables = renderer.VisualVariables?.ToList() ?? new List<CIMVisualVariable>(); if (vis_variables.Count == 0) return;//there are none var vis_var_with_expr = new Dictionary<string, string>(); //see if any are using expressions foreach (var vv in vis_variables) { if (vv is CIMColorVisualVariable cvv) { if (!string.IsNullOrEmpty(cvv.ValueExpressionInfo?.Expression)) vis_var_with_expr.Add("Color", cvv.ValueExpressionInfo?.Expression); } else if (vv is CIMTransparencyVisualVariable tvv) { if (!string.IsNullOrEmpty(tvv.ValueExpressionInfo?.Expression)) vis_var_with_expr.Add("Transparency", tvv.ValueExpressionInfo?.Expression); } else if (vv is CIMSizeVisualVariable svv) { if (!string.IsNullOrEmpty(svv.ValueExpressionInfo?.Expression)) vis_var_with_expr.Add("Outline", svv.ValueExpressionInfo?.Expression); } } if (vis_var_with_expr.Count == 0) return;//there arent any with expressions //loop through the features (outer) //per feature evaluate each visual variable.... (inner) //.... //the converse is to loop through the expressions (outer) //then per feature evaluate the expression (inner) using (var rc = oregon_cnts.Search()) { while (rc.MoveNext()) { foreach (var kvp in vis_var_with_expr) { var expr_info = new CIMExpressionInfo() { Expression = kvp.Value, ReturnType = ExpressionReturnType.Default }; //per feature eval each expression... using (var arcade = ArcadeScriptEngine.Instance.CreateEvaluator( expr_info, ArcadeProfile.Visualization)) { var variables = new List<KeyValuePair<string, object>>() { new KeyValuePair<string, object>("$feature", rc.Current) }; //note 2D maps can also have view scale... //...if necessary... if (mv.ViewingMode == MapViewingMode.Map) { variables.Add(new KeyValuePair<string, object>( "$view.scale", mv.Camera.Scale)); } var result = arcade.Evaluate(variables).GetResult().ToString(); //output System.Diagnostics.Debug.WriteLine( $"[{rc.Current.GetObjectID()}] '{kvp.Key}': {result}"); } } } } ////foreach (var kvp in vis_var_with_expr) ////{ //// var expr_info = new CIMExpressionInfo() //// { //// Expression = kvp.Value, //// ReturnType = ExpressionReturnType.Default //// }; //// using (var arcade = ArcadeScriptEngine.Instance.CreateEvaluator( //// expr_info, ArcadeProfile.Visualization)) //// { //// //loop through the features //// using (var rc = oregon_cnts.Search()) //// { //// while (rc.MoveNext()) //// { //// var variables = new List<KeyValuePair<string, object>>() { //// new KeyValuePair<string, object>("$feature", rc.Current) //// }; //// var result = arcade.Evaluate(variables).GetResult(); //// //output //// //... //// } //// } //// } ////} });
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMVisualVariable
ArcGIS.Core.CIM.CIMColorVisualVariable
ArcGIS.Core.CIM.CIMColorClassBreaksVisualVariable
Target Platforms: Windows 11, Windows 10